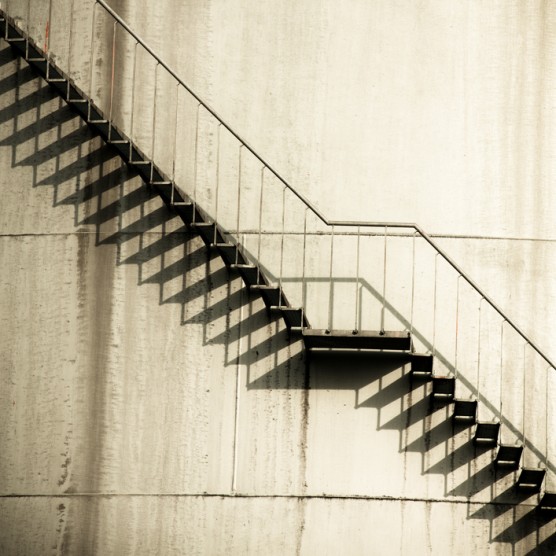
Querying the JIRA api with Meteor
For anyone trying to figure out how to connect to the JIRA api with Meteor JS: I was using the POST call with my JIRA credentials (as the POST data) and url=https://company.atlassian.net/rest/auth/latest/session to get the session cookie that I could use for authentication in the later API calls.
The login call above returns an array cookies (with session name and values, atlassian.xsrf.token, studio.crowd.tokenkey):
It turns out that the cookie #3 is the one causing the problem. To get the API calls working I parse through the returned cookie array and delete the one with expiration date in it and then I pass the entire array of cookies.
Example code:
Note: Thanks to Dan Dascalescu from The Quantified Self Forum for catching an input filter issue resulting in a javascript error in the code above!
The login call above returns an array cookies (with session name and values, atlassian.xsrf.token, studio.crowd.tokenkey):
- "atlassian.xsrf.token=BZD0-ZZ0R-LNEL-XXXX|fb1e90160161c98de5d2b5086xxxxxxxxxxxxxxxx|lout; Path=/"
- "JSESSIONID=0BB474286C3F6356013XXXXXXXXX; Path=/; HttpOnly"
- "studio.crowd.tokenkey=""; Domain=.company.atlassian.net; Expires=Thu, 01-Jan-1970 00:00:10 GMT; Path=/; HttpOnly"
- "studio.crowd.tokenkey=jJv5rU3Fs60A8VaIgXXXXXX; Domain=.projectricochet.atlassian.net; Path=/; HttpOnly"
It turns out that the cookie #3 is the one causing the problem. To get the API calls working I parse through the returned cookie array and delete the one with expiration date in it and then I pass the entire array of cookies.
Example code:
var auth_url="https://company.atlassian.net/rest/auth/latest/session"; var result = Meteor.http.call("POST", auth_url, { data: { 'username': ‘admin’, 'password': ‘admin’, }, headers: { "content-type": "application/json", "Accept": "application/json" }, }); var cookie_value = result['headers']['set-cookie']; for(var c in cookie_value) { if(cookie_value[c].indexOf("Expires") !== -1) { cookie_value[c] = ""; } } if (result.statusCode == 200) { var auth_url="https://company.atlassian.net/rest/api/latest/issue/ISSUE#"; result = Meteor.http.call("GET", auth_url, { params: { timeout: 30000 }, headers: { "cookie": cookie_value, "content-type": "application/json", "Accept": "application/json" }, }); var issue = JSON.parse(result.content); console.log(issue); }
Note: Thanks to Dan Dascalescu from The Quantified Self Forum for catching an input filter issue resulting in a javascript error in the code above!